在Vue3中使用Websocket可以讓我們輕松地實現(xiàn)實時數(shù)據(jù)傳輸。為了方便使用,我們可以封裝一個好用的Websocket類。
安裝依賴
首先我們需要安裝 ws 庫來處理Websocket連接,使用以下命令進行安裝:
npm install ws --save
封裝Websocket類
我們可以新建一個 websocket.js 文件,在其中定義一個 Websocket 類,代碼如下:
import WebSocket from 'ws';
class Websocket {
constructor(url, options = {}) {
this.url = url;
this.options = options;
this.ws = null;
}
connect() {
this.ws = new WebSocket(this.url, this.options);
this.ws.onopen = () => {
console.log('Websocket connection opened.');
};
this.ws.onmessage = (event) => {
console.log('Websocket message received.', event.data);
};
this.ws.onerror = (error) => {
console.error('Websocket error occurred.', error);
};
this.ws.onclose = () => {
console.log('Websocket connection closed.');
};
}
send(data) {
if (this.ws.readyState === WebSocket.OPEN) {
this.ws.send(data);
} else {
console.error('Websocket connection not open.');
}
}
close() {
this.ws.close();
}
}
export default Websocket;
以上代碼中,我們定義了一個 Websocket 類,其中包含了 connect 方法用于連接Websocket服務器, send 方法用于發(fā)送數(shù)據(jù), close 方法用于關閉連接。
在Vue3中使用Websocket
在Vue3中,我們可以將Websocket類封裝成一個Vue插件,以便全局使用。示例代碼如下:
import Websocket from './websocket.js';
const MyPlugin = {
install(Vue) {
Vue.prototype.$websocket = new Websocket('ws://localhost:8080');
},
};
export default MyPlugin;
在 main.js 文件中我們可以使用 Vue.use 方法來使用插件:
import { createApp } from 'vue';
import App from './App.vue';
import MyPlugin from './my-plugin.js';
const app = createApp(App);
app.use(MyPlugin);
app.mount('#app');
現(xiàn)在我們就可以在Vue3組件中使用 $websocket 對象,例如:
export default {
mounted() {
this.$websocket.connect();
},
methods: {
sendMessage(message) {
this.$websocket.send(message);
},
},
};
總結(jié)
通過封裝Websocket類,我們可以在Vue3中輕松使用Websocket進行實時數(shù)據(jù)傳輸。
安裝依賴
首先我們需要安裝 ws 庫來處理Websocket連接,使用以下命令進行安裝:
npm install ws --save
封裝Websocket類
我們可以新建一個 websocket.js 文件,在其中定義一個 Websocket 類,代碼如下:
import WebSocket from 'ws';
class Websocket {
constructor(url, options = {}) {
this.url = url;
this.options = options;
this.ws = null;
}
connect() {
this.ws = new WebSocket(this.url, this.options);
this.ws.onopen = () => {
console.log('Websocket connection opened.');
};
this.ws.onmessage = (event) => {
console.log('Websocket message received.', event.data);
};
this.ws.onerror = (error) => {
console.error('Websocket error occurred.', error);
};
this.ws.onclose = () => {
console.log('Websocket connection closed.');
};
}
send(data) {
if (this.ws.readyState === WebSocket.OPEN) {
this.ws.send(data);
} else {
console.error('Websocket connection not open.');
}
}
close() {
this.ws.close();
}
}
export default Websocket;
以上代碼中,我們定義了一個 Websocket 類,其中包含了 connect 方法用于連接Websocket服務器, send 方法用于發(fā)送數(shù)據(jù), close 方法用于關閉連接。
在Vue3中使用Websocket
在Vue3中,我們可以將Websocket類封裝成一個Vue插件,以便全局使用。示例代碼如下:
import Websocket from './websocket.js';
const MyPlugin = {
install(Vue) {
Vue.prototype.$websocket = new Websocket('ws://localhost:8080');
},
};
export default MyPlugin;
在 main.js 文件中我們可以使用 Vue.use 方法來使用插件:
import { createApp } from 'vue';
import App from './App.vue';
import MyPlugin from './my-plugin.js';
const app = createApp(App);
app.use(MyPlugin);
app.mount('#app');
現(xiàn)在我們就可以在Vue3組件中使用 $websocket 對象,例如:
export default {
mounted() {
this.$websocket.connect();
},
methods: {
sendMessage(message) {
this.$websocket.send(message);
},
},
};
總結(jié)
通過封裝Websocket類,我們可以在Vue3中輕松使用Websocket進行實時數(shù)據(jù)傳輸。
付費1元即可閱讀全文
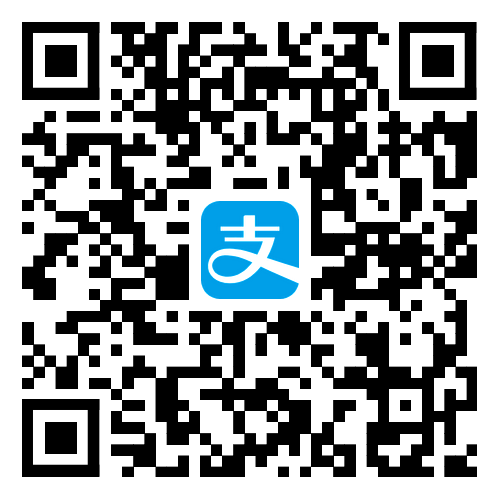
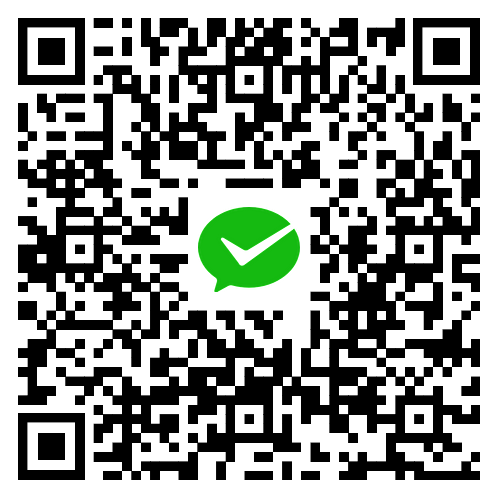